Often, applications use environmental variables to store secrets or values that vary depending on the system where the application runs. These values are typically not checked into the git repositories, and you may need to set them at runtime. The question then arises as to where to save these values so that they are available to your application at runtime.
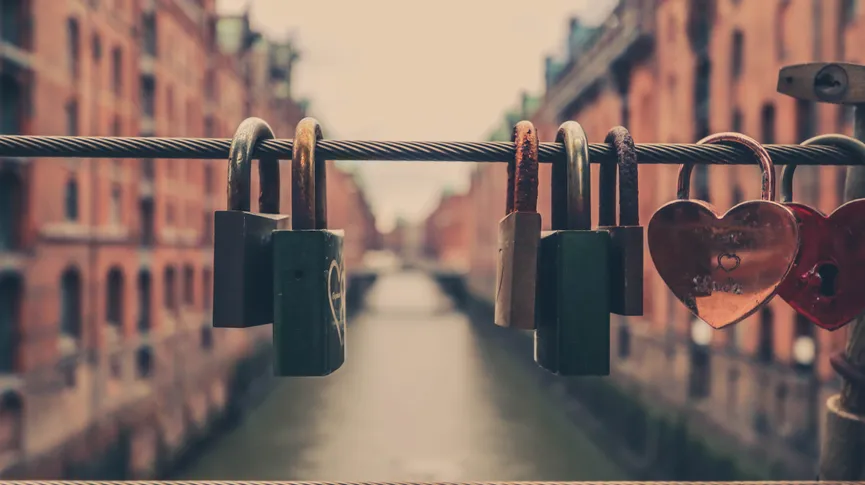
One option is to store them in AWS Secrets Manager and use the AWS SDK to fetch them during runtime. In NestJS, you can easily load these values into the ConfigService
.
To begin, ensure that the machine running the NestJS application has the necessary permissions to access the AWS Secrets Manager resource. Then, use the following code to fetch the secret values:
import { SecretsManager } from "@aws-sdk/client-secrets-manager";
export default async (): Promise<Record<string, string>> => {
const secretsManager = new SecretsManager({});
try {
const data = await secretsManager.getSecretValue({ SecretId: "my-app/secrets" });
if (data.SecretString) {
const secret = JSON.parse(data.SecretString);
return secret as Record<string, string>;
}
} catch (err) {
console.error(err);
}
return {};
};
Finally, add the secrets to the ConfigModule
within the app.module.ts
file:
@Module({
imports: [ConfigModule.forRoot({ isGlobal: true, load: [secrets] })],
})
export class AppModule {}
With these steps, your application will fetch the secret key value data from AWS Secrets Manager and load it into the ConfigService at runtime.
In conclusion, storing sensitive information like passwords or API keys in environmental variables can be a security risk. AWS Secrets Manager provides a secure and easy-to-use solution for storing and managing such sensitive data. With the help of the AWS SDK and the NestJS ConfigService
, it is possible to fetch the secrets from AWS Secrets Manager and load them into your application at runtime. This approach ensures that your sensitive information remains secure while still being easily accessible to your application.