RXJava can be very intimidating at first go. Reactive Extensions - RX is all about data streams & handling them and three important pillars for it are Observables, Operators & Schedulers.
Initially, while using operators I was very confused between map and flatMap. And this confusion still exists today with all beginners. So let me help you to clear this confusion.
Map
Map operator transforms the items from data stream by applying a function to each item. Lets look at below code which will output length of words.
Example:
String line = "Lorem Ipsum is simply dummy text";
List words = Arrays.asList(line.split(" "));
Observable.from(words)
.map(w -> {
return String.format("%s => %s", w, w.length());
}).subscribe(System.out::println);
Here we are passing list of words to map. For each word it formats it and return back to the stream.
Marble Diagram:
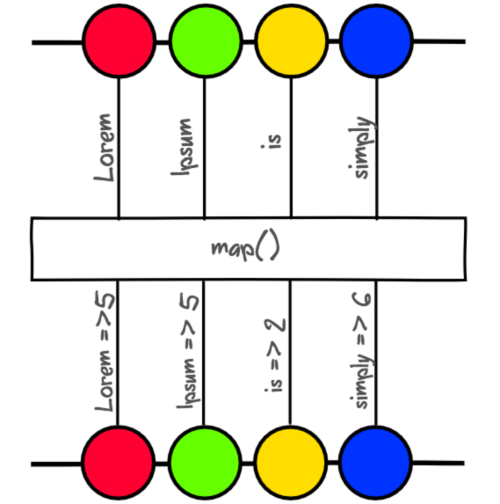
FlatMap
FlatMap operator transforms the items from data stream into observables and then it flattens all observables into single observable. Lets look at below code which will output letters from words.
String line = "Lorem Ipsum is simply dummy text";
List words = Arrays.asList(line.split(" "));
Observable.from(words)
.flatMap(w -> {
return Observable.from(w.split(""));
}).subscribe(System.out::println);
Confused??
In map when word Lorem
was passed, it transformed it into Lorem => 5
. But when we passed same word to flatMap, it transformed it into ["L","o","r","e","m"]
. So flatMap can emit one or more than one values whereas map emits only one. Because its an array, flatMap flattens all values in a single stream.
Marble Diagram:
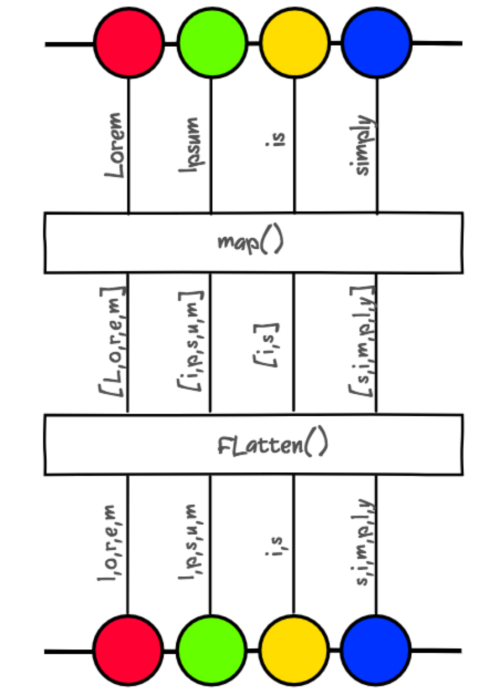